Java cung cấp ba cách để tạo số ngẫu nhiên bằng cách sử dụng một số phương thức và lớp tích hợp như được liệt kê bên dưới:
- lớp java.util.Random
- Phương pháp Math.random: Có thể tạo số ngẫu nhiên kiểu kép.
- Lớp ThreadLocalRandom
1) java.util.Random
Để sử dụng lớp này để tạo radom trong java, trước tiên chúng ta phải tạo một thể hiện của lớp này và sau đó gọi các phương thức như nextInt (), nextDouble (), nextLong (), v.v. bằng cách sử dụng thể hiện đó.
Chúng ta có thể tạo số ngẫu nhiên kiểu số nguyên, float, double, long, boolean bằng cách sử dụng lớp này.
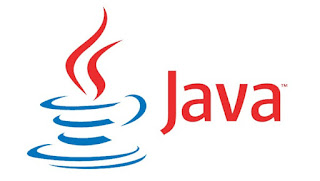
Chúng ta có thể truyền các đối số cho các phương thức để đặt giới hạn trên trên phạm vi số sẽ được tạo. Ví dụ: nextInt (6) sẽ tạo ra các số trong phạm vi từ 0 đến 5 bao gồm cả hai.
public class generateRandom{
public static void main(String args[])
{
// create instance of Random class
Random rand = new Random();
// Generate random integers in range 0 to 999
int rand_int1 = rand.nextInt(1000);
int rand_int2 = rand.nextInt(1000);
// Print random integers
System.out.println("Random Integers: "+rand_int1);
System.out.println("Random Integers: "+rand_int2);
// Generate Random doubles
double rand_dub1 = rand.nextDouble();
double rand_dub2 = rand.nextDouble();
// Print random doubles
System.out.println("Random Doubles: "+rand_dub1);
System.out.println("Random Doubles: "+rand_dub2);
}
}
Kết quả:
Random Integers: 547
Random Integers: 126
Random Doubles: 0.8369779739988428
Random Doubles: 0.5497554388209912
2) Math.random()
Lớp Math chứa nhiều phương thức khác nhau để thực hiện các phép toán số khác nhau như, tính lũy thừa, logarit, v.v. Một trong những phương thức này là random () java, phương thức này trả về giá trị kép với dấu dương, lớn hơn hoặc bằng 0,0 và nhỏ hơn 1,0 . Các giá trị trả về được chọn không thường xuyên. Phương pháp này chỉ có thể tạo ra các số ngẫu nhiên kiểu Đôi. Chương trình dưới đây giải thích cách sử dụng phương pháp này:
public class generateRandom
{
public static void main(String args[])
{
// Generating random doubles
System.out.println("Random doubles: " + Math.random());
System.out.println("Random doubles: " + Math.random());
}
}
Kết quả:
Random doubles: 0.13077348615666562
Random doubles: 0.09247016928442775
3) java.util.concurrent.ThreadLocalRandom class
Lớp này được giới thiệu trong java 1.7 để tạo các số ngẫu nhiên kiểu số nguyên, đôi, boolean, v.v. Chương trình dưới đây giải thích cách sử dụng lớp này để tạo số ngẫu nhiên
public class generateRandom
{
public static void main(String args[])
{
// Generate random integers in range 0 to 999
int rand_int1 = ThreadLocalRandom.current().nextInt();
int rand_int2 = ThreadLocalRandom.current().nextInt();
// Print random integers
System.out.println("Random Integers: " + rand_int1);
System.out.println("Random Integers: " + rand_int2);
// Generate Random doubles
double rand_dub1 = ThreadLocalRandom.current().nextDouble();
double rand_dub2 = ThreadLocalRandom.current().nextDouble();
// Print random doubles
System.out.println("Random Doubles: " + rand_dub1);
System.out.println("Random Doubles: " + rand_dub2);
// Generate random booleans
boolean rand_bool1 = ThreadLocalRandom.current().nextBoolean();
boolean rand_bool2 = ThreadLocalRandom.current().nextBoolean();
// Print random Booleans
System.out.println("Random Booleans: " + rand_bool1);
System.out.println("Random Booleans: " + rand_bool2);
}
}
Kết quả:
Random Integers: 536953314
Random Integers: 25905330
Random Doubles: 0.7504989954390163
Random Doubles: 0.7658597196204409
Random Booleans: false
Random Booleans: true
No comments:
Post a Comment